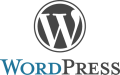
When you schedule a post, if your WordPress theme displays both published date and modified date, you will see that the modified date is older than the published date. It can look odd because normally, modified or updated date should be later than the published date.
In this article, I will show you the codes that can fix the problem.
Showing both published date and modified date
To display the published date in a blog post, you can insert the_time()
in your theme's single.php or loop.php. And to display the modified date, you can insert the_modified_time()
.
Initially, my theme had these codes in its single.php file to display both dates:
Published on <?php the_time('j F Y'); ?>. Last updated on <?php the_modified_time('j F Y'); ?>.
The 'j F Y'
inside the brackets are to set the date format following the PHP DateTime format. j
to show the day of the month without leading zeros, F
to show full name of the month, and Y
to show the year in four digits. So, the dates will be displayed in the format like 5 July 2022.
Problem with dates in a scheduled post
Let's say you have finished writing a post on 5 July 2022 and you scheduled it to be published on 2 August 2022.
If your theme's single.php has the codes above, your scheduled post will show: "Published on 2 August 2022. Last updated on 5 July 2022".
The workaround
To solve that problem, we can add conditional statements. For that, we need to compare both dates first to find which one is the older date.
Comparing dates in WordPress
To compare modified date and published date, we use get_the_modified_date()
and get_the_date()
functions.
The date format should use 'Y m d'
so everything will be in number and the order goes from year, month, to date. For example, 5 July 2022 will be outputted as 20220705 and 2 August 2022 will be 20220802. This date format will make it easier to compare dates.
So, if you want to compare the dates, you can use this if else statement:
if (get_the_modified_date('Y m d') <= get_the_date('Y m d')) {
code to be executed if the modified date is earlier than or the same as the published date
} else {
code to be executed if the modified date is later than the published date
}
The application examples
Now let's put the code into examples. This code can be inserted to WordPress theme's single.php and loop.php. It may need some adjustments depending on the theme you are using.
Example 1
The goal:
If the modified date is older than or equal to the published date, only show the published date. If the modified date is later than the published date, only show the modified date.
The code:
if (get_the_modified_date('Y m d') <= get_the_date('Y m d')) {
echo 'Published on ' . get_the_date('j F Y') . '.';
} else {
echo 'Last updated on ' . get_the_modified_date('j F Y') . '.';
}
Example 2
The goal:
Always show the published date. If the modified date is later than the published date, add / show the modified date as well.
The code:
Published on <?php the_time('j F Y'); ?>.<?php if (get_the_modified_date('Y m d') > get_the_date('Y m d')): ?> Last updated on <?php the_modified_time('j F Y');?>.<?php endif; ?>
I hope this post can help you. Happy coding! 🙂